Amazon Simple Queue Service (SQS) is a message queue service that allows applications to reliably queue messages from one system component to be consumed by another component. Adding a queue between application components allows them to run independently, effectively decoupling applications by providing a buffer between producers of data and their consumers.
Up and Running
This section provides a guide to getting up and running with SQS using a fictional music store as an example. This music store has the ability to notify clients of any upcoming events via SQS.
In SQS have a pre-defined life cycle. First, application A adds a message to a queue. SQS redundantly stores this message across the SQS servers. When application B is ready, it pulls the message from the queue and processes it. The act of pulling the message triggers a visibility timeout in SQS. During this timeout period, no other consumers are allowed to pull that message from the queue. After application B has fully processed the message, it deletes the message from the queue. This process ensures that at most one consumer processes each message in the queue.
The following few examples show how to manage this life cycle to create, consume, and delete messages.
Creating a Queue
All interactions with SQS involve a queue. This can be done
programmatically or through the SQS user interface. To follow along with
this tutorial, create a queue through the user interface. Call that queue
MusicStore
.
After creating your queue, take the time to note two configuration options: (1) the AWS region of the queue, and (2) the queues URL. We will need this information to send messages a message to our queue.
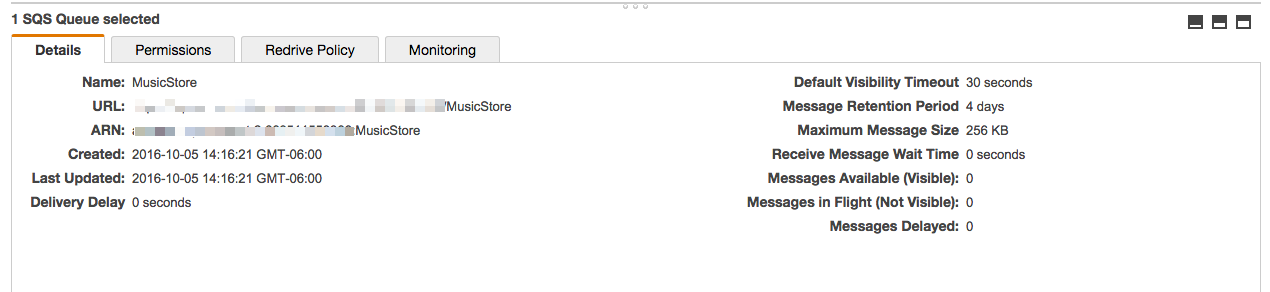
Sending a Message
Although you can send a message using the SQS user interface, this task is usually done programmatically. Thankfully, if you know the queue you want to use, sending a message to it is a fairly simple operation.
package com.sookocheff.sqs;
import com.amazonaws.auth.AWSCredentials;
import com.amazonaws.auth.DefaultAWSCredentialsProviderChain;
import com.amazonaws.regions.Region;
import com.amazonaws.regions.Regions;
import com.amazonaws.services.sqs.AmazonSQS;
import com.amazonaws.services.sqs.AmazonSQSClient;
import com.amazonaws.services.sqs.model.SendMessageRequest;
/**
* Demo for sending a message using SQS.
*/
public class MessageSender {
public static void main(String[] args) throws Exception {
// Load your AWS credentials
AWSCredentials credentials = new DefaultAWSCredentialsProviderChain().getCredentials();
Region usWest2 = Region.getRegion(Regions.US_WEST_2);
// Create an SQS client
AmazonSQS sqs = new AmazonSQSClient(credentials);
sqs.setRegion(usWest2);
// Send a message
System.out.println("Sending a message to MusicStore.\n");
sqs.sendMessage(
new SendMessageRequest()
.withQueueUrl("https://sqs.us-west-2.amazonaws.com/****/MusicStore")
.withMessageBody("The Lumineers coming this weekend!"));
}
}
The preceding code begins by loading your AWS credentials, then creating
a new SQS client configured with the us-west-2 AWS region. Finally, you
send a message using the SQS client and a SendMessageRequest
.
Receiving a Message
The message you sent is available in your SQS queue. You can verify this by navigating to the SQS dashboard on the AWS console.

Knowing the SQS queue URL and region, receiving a message is equally simple.
package com.sookocheff.sqs;
import com.amazonaws.auth.AWSCredentials;
import com.amazonaws.auth.DefaultAWSCredentialsProviderChain;
import com.amazonaws.regions.Region;
import com.amazonaws.regions.Regions;
import com.amazonaws.services.sqs.AmazonSQS;
import com.amazonaws.services.sqs.AmazonSQSClient;
import com.amazonaws.services.sqs.model.Message;
import com.amazonaws.services.sqs.model.ReceiveMessageRequest;
import com.amazonaws.services.sqs.model.ReceiveMessageResult;
/**
* Simple SQS message receiver.
*/
public class MessageReceiver {
public static void main(String[] args) throws Exception {
// Load your AWS credentials
AWSCredentials credentials = new DefaultAWSCredentialsProviderChain().getCredentials();
Region usWest2 = Region.getRegion(Regions.US_WEST_2);
// Create an SQS client
AmazonSQS sqs = new AmazonSQSClient(credentials);
sqs.setRegion(usWest2);
// Receive a message
System.out.println("Receiving a message to MusicStore.\n");
ReceiveMessageResult messageResult = sqs.receiveMessage(
new ReceiveMessageRequest()
.withQueueUrl("https://sqs.us-west-2.amazonaws.com/****/MusicStore"));
for (Message message : messageResult.getMessages()) {
System.out.println("Received: " + message.getBody());
}
}
}
Delete a Message
You can run the simple program above again and again to receive the same message. With SQS, messages are not removed from the queue unless they are explicitly deleted. This mechanism ensures the messages are not removed from the queue until they have been successfully consumed.
The following sample program shows how to delete a message, removing it
from the queue and ensuring no other consumers will process it. To delete
a message requires access to the messages identifier, which is available
the messages receipt handle via the getReceiptHandle
method.
package com.sookocheff.sqs;
import com.amazonaws.auth.AWSCredentials;
import com.amazonaws.auth.DefaultAWSCredentialsProviderChain;
import com.amazonaws.regions.Region;
import com.amazonaws.regions.Regions;
import com.amazonaws.services.sqs.AmazonSQS;
import com.amazonaws.services.sqs.AmazonSQSClient;
import com.amazonaws.services.sqs.model.DeleteMessageRequest;
import com.amazonaws.services.sqs.model.Message;
import com.amazonaws.services.sqs.model.ReceiveMessageRequest;
import com.amazonaws.services.sqs.model.ReceiveMessageResult;
/**
* Simple SQS message deleter.
*/
public class MessageDeleter {
public static void main(String[] args) throws Exception {
// Load your AWS credentials
AWSCredentials credentials = new DefaultAWSCredentialsProviderChain().getCredentials();
Region usWest2 = Region.getRegion(Regions.US_WEST_2);
// Create an SQS client
AmazonSQS sqs = new AmazonSQSClient(credentials);
sqs.setRegion(usWest2);
// Receive a message (to get the messages metadata)
ReceiveMessageResult messageResult = sqs.receiveMessage(
new ReceiveMessageRequest()
.withQueueUrl("https://sqs.us-west-2.amazonaws.com/****/MusicStore"));
// Delete a message
Message message = messageResult.getMessages().get(0);
sqs.deleteMessage(new DeleteMessageRequest()
.withQueueUrl("https://sqs.us-west-2.amazonaws.com/****/MusicStore")
.withReceiptHandle(message.getReceiptHandle()));
}
}